Microservices have transformed software development, making applications more scalable, resilient, and flexible.
However, relying on synchronous communication (REST API calls) between services can introduce latency, tight coupling, and failure points.
Enter Event-Driven Architecture (EDA)—a powerful approach that decouples services and allows them to communicate asynchronously through events.
In this guide, we’ll explore how to implement an event-driven microservices architecture in Spring Boot using Kafka, RabbitMQ, and Spring Cloud Stream, with real-world use cases such as financial transactions, real-time notifications, and messaging systems.
What is Event-Driven Architecture?
Event-driven architecture (EDA) is a design pattern where services communicate via events rather than direct API calls. Services produce and consume events asynchronously, reducing dependencies and improving performance.
Key Benefits
✔ Loose Coupling – Services operate independently.
✔ Scalability – Events can be processed in parallel.
✔ Resilience – Failures in one service do not break the entire system.
✔ Real-Time Processing – Ideal for financial transactions, notifications, and analytics.
Real-World Use Cases 🎯
- Financial Transactions: A Teller Cash Deposit event updates accounting and notification services.
- Real-Time Notifications: A New Transaction Event triggers WebSocket-based alerts.
- Audit Logging: A Transaction Completed Event ensures compliance tracking asynchronously.
My Experience: Using Event-Driven Architecture
I’m currently working on a microservices-based banking solution designed to streamline financial transactions. Built using Spring Boot and Java 17, it ensures secure and efficient handling of various teller operations. The system is designed for scalability and modularity, allowing seamless integration with external banking gateways.
By implementing event-driven architecture, I transformed how financial transactions are processed. Using Apache Kafka and RabbitMQ, I ensured that transactions, notifications, and reconciliations were handled asynchronously, leading to:
✅ Faster transaction processing – No blocking requests.
✅ Improved resilience – Failures in one microservice do not disrupt others.
✅ Scalability – Services process events in parallel, handling high transaction loads.
✅ Better user experience – Real-time notifications inform users instantly.
For example, when a teller cash deposit occurs:
1️⃣ A deposit event is published to Kafka.
2️⃣ The Accounting Service updates the balance asynchronously.
3️⃣ The Notification Service sends real-time alerts.
4️⃣ The Audit Service logs the transaction.
This approach eliminated bottlenecks, reduced API dependencies, and ensured the system could handle high transaction volumes efficiently.
Step 1: Choosing an Event Broker
For Java microservices, the most popular event brokers are:
Apache Kafka– Best for high-throughput, distributed messaging.
RabbitMQ– Best for lightweight, message-queuing use cases.
Spring Cloud Stream – Abstracts messaging brokers, making it easy to switch between Kafka and RabbitMQ.
Step 2: Implementing Kafka Producer
Here’s how to publish events using Apache Kafka in Spring Boot:
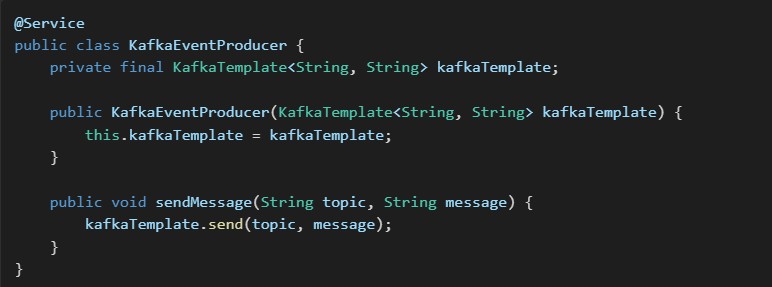
Step 3: Implementing Kafka Consumer
To consume events from Kafka:
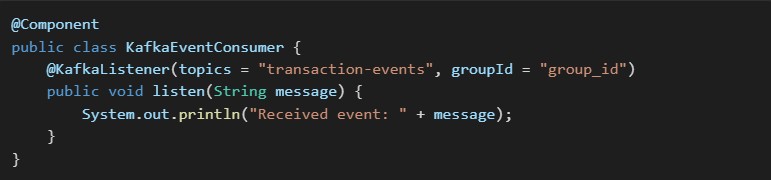
Step 4: Implementing RabbitMQ Producer
To publish events using RabbitMQ:
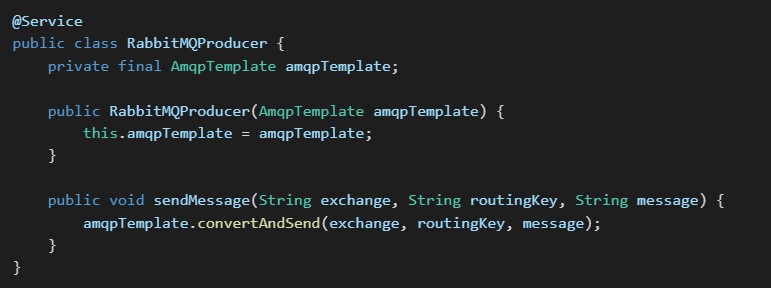
Step 5: Implementing RabbitMQ Consumer
To consume messages from RabbitMQ:
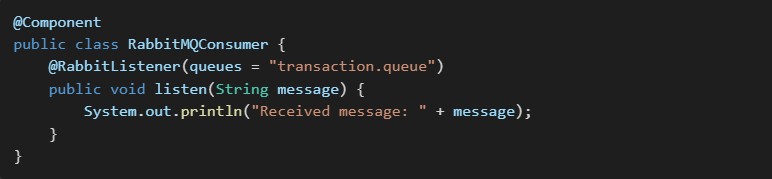
Step 6: Event-Driven Processing with Spring Cloud Stream
Spring Cloud Stream simplifies event-driven processing by abstracting messaging brokers.
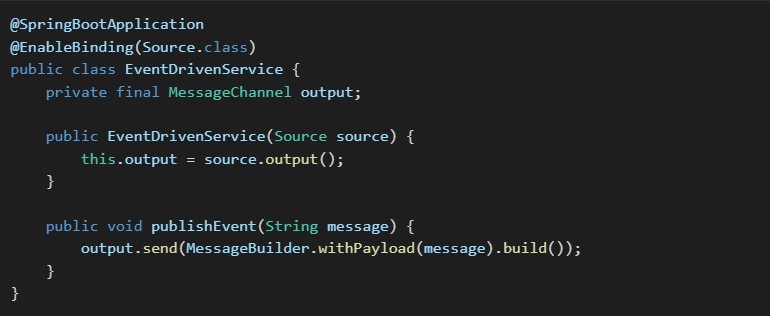
Conclusion
Event-Driven Architecture is a game-changer for microservices scalability, resilience, and real-time processing.
🔹 Kafka – Best for high-throughput streaming (e.g., financial transactions).
🔹 RabbitMQ – Best for lightweight messaging (e.g., user notifications).
🔹 Spring Cloud Stream – Best for flexibility across multiple brokers.
By integrating event-driven principles into my project, I have built a highly scalable, decoupled architecture that ensures reliable, real-time transaction processing.
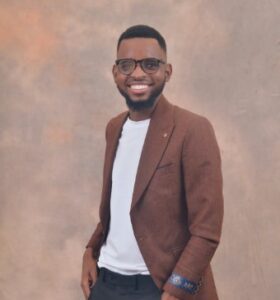
Tafadzwa Allen Pundo
Senior Software Engineer at Skywalk Innovations