In today’s software landscape, achieving high performance and scalability is a necessity for modern applications.
Leveraging powerful tools like Redis alongside the robust Spring Boot framework can help developers meet these demands. If you’re interested in integrating Redis with Spring Boot effectively, flanchoooo’s Spring Redis Implementation repository offers a fantastic starting point.
This blog will guide you through key features of this implementation and highlight how you can use it to build scalable, cache-optimized applications.
Why Redis?
Redis is an in-memory data structure store known for its:
– Speed: Redis operates in memory, making it extremely fast for reads and writes.
– Versatility: Supports data structures like strings, hashes, lists, sets, and more.
– Scalability: Built-in replication and clustering enable horizontal scalability.
– Use Cases: Commonly used for caching, session storage, leaderboards, and real-time analytics.
When combined with Spring Boot, Redis becomes an excellent choice for implementing caching,
distributed locks, or real-time processing systems.
About the Spring Redis Implementation
The repository demonstrates how to integrate Redis into a Spring Boot application. Key features include:
1. Caching with Redis
Caching is one of the primary use cases for Redis. The implementation uses Spring’s @Cacheable, @CacheEvict, and @CachePut annotations to manage caching effectively. By doing so, applications can reduce database load and improve response times.
Example Configuration

This method caches the result of fetching an item by ID. Subsequent calls with the same ID will retrieve the value from Redis instead of querying the database.
2. Redis Configuration
The repository demonstrates how to configure a Redis connection using application.properties or
application.yml. Typical configurations include:
– Redis host and port.
– Connection pool settings.
– Serialization strategies for storing and retrieving objects
Example Configuration
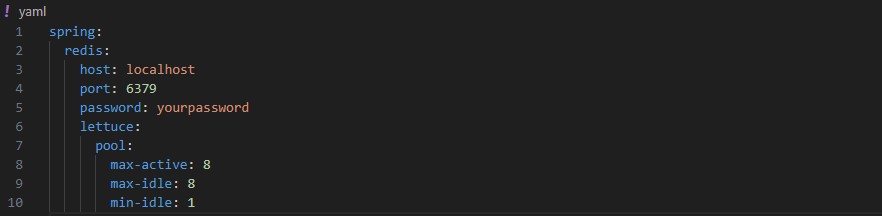
3. Serialization Strategies
Redis stores data as byte streams, requiring proper serialization and deserialization. This
implementation uses JSON (via Jackson) or other formats for handling complex objects.
Code Example:

4. Distributed Locking
Redis can act as a simple and effective distributed lock mechanism. This repository showcases how
to use Redis to handle scenarios where multiple instances of an application need to coordinate
access to shared resources.
How to Use the Repository
1. Clone the Repository
git clone https://github.com/flanchoooo/spring-redis-impl.git
cd spring-redis-impl
2. Set Up Redis
– Install Redis on your system or use a cloud-hosted Redis service like AWS Elasticache or Azure
Redis Cache.
– Update the connection details in application.properties.
3. Run the Application
Use Maven or Gradle to build and run the application:
./mvnw spring-boot:run
4. Experiment with Features
Explore caching, session storage, or distributed locking using the examples provided in the
repository.
Extending the Implementation
This repository provides a foundation that you can extend for specific use cases. Here are some
ideas:
1. Session Management: Use Redis to manage user sessions in a stateless architecture.
2. Pub/Sub Messaging: Leverage Redis’ publish/subscribe feature to implement event-driven
microservices.
3. Data Expiry: Define time-to-live (TTL) for cached data to ensure stale data doesn’t persist indefinitely.
4. Clustering and Replication: Scale your Redis infrastructure for high availability.
Conclusion
Integrating Redis with Spring Boot can significantly enhance your application’s performance and scalability. The Spring Redis Implementation repository simplifies this process by providing practical examples of caching, configuration, and more. Whether you’re building a simple caching layer or a robust distributed system, Redis and Spring Boot together offer the tools you need to succeed.
Check out the repository and start optimizing your application today!
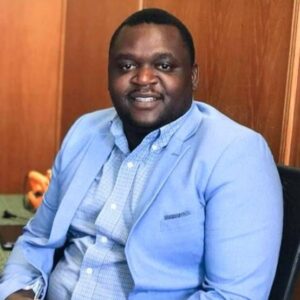
Flavian Machimbirike
Senior Software Engineer at Skywalk Innovations